TIL Poor man's quoting in Python
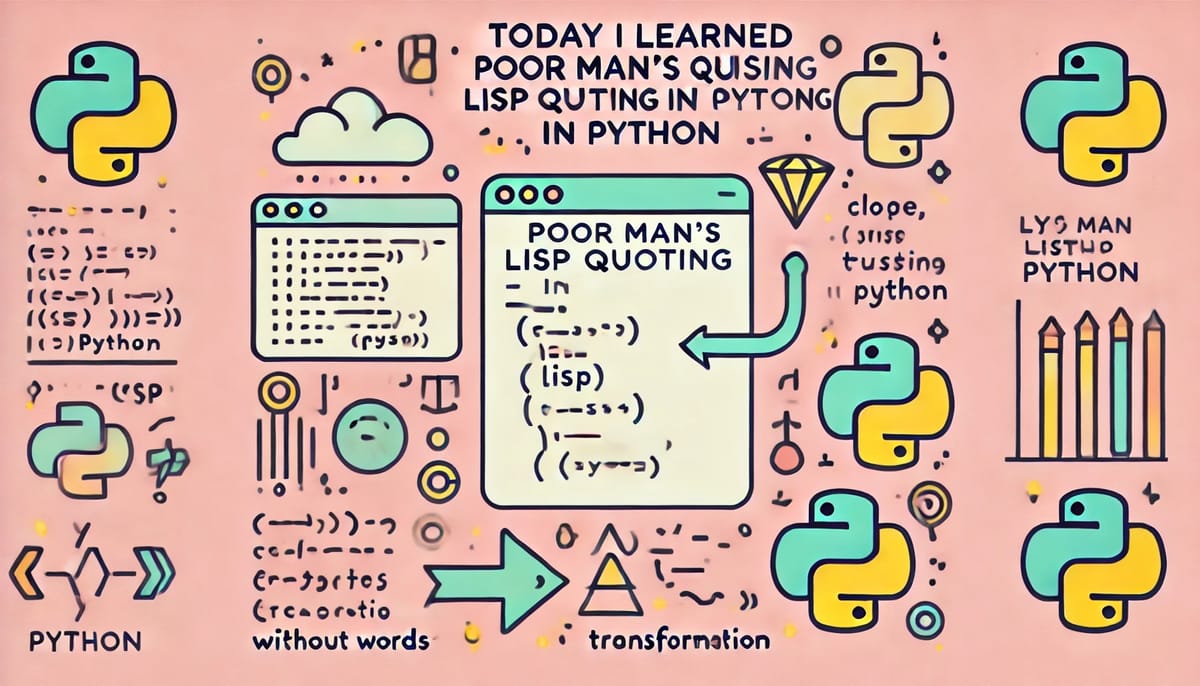
In Lisp, you can quote an expression to delay its evaluation while passing it around as a value. In Python, this means in practice, I want a function that looks like it's executing, but reality, I'm delaying its actual execution.
I can use decorators to achieve this.
# A thunk-based Efx decorator
def Efx(func):
@wraps(func)
def thunk(*children, **args):
args['children'] = children
return lambda: func(*children, **args)
return thunk
And then I can use it like so:
@Efx
def SomeComponent(*children, **args):
return ComponentA(
ComponentB(propA = args.propA)
)
thunk = SomeComponent(propA = "hello")
# The component doesn't get executed until we call the thunk.
thunk() = # some dict representation of components
The @wraps
decorator transfers the metadata of the function, so that we can query SomeComponent()
's metadata, even though it's wrapped in a decorator.