Explain a Kleisli Category
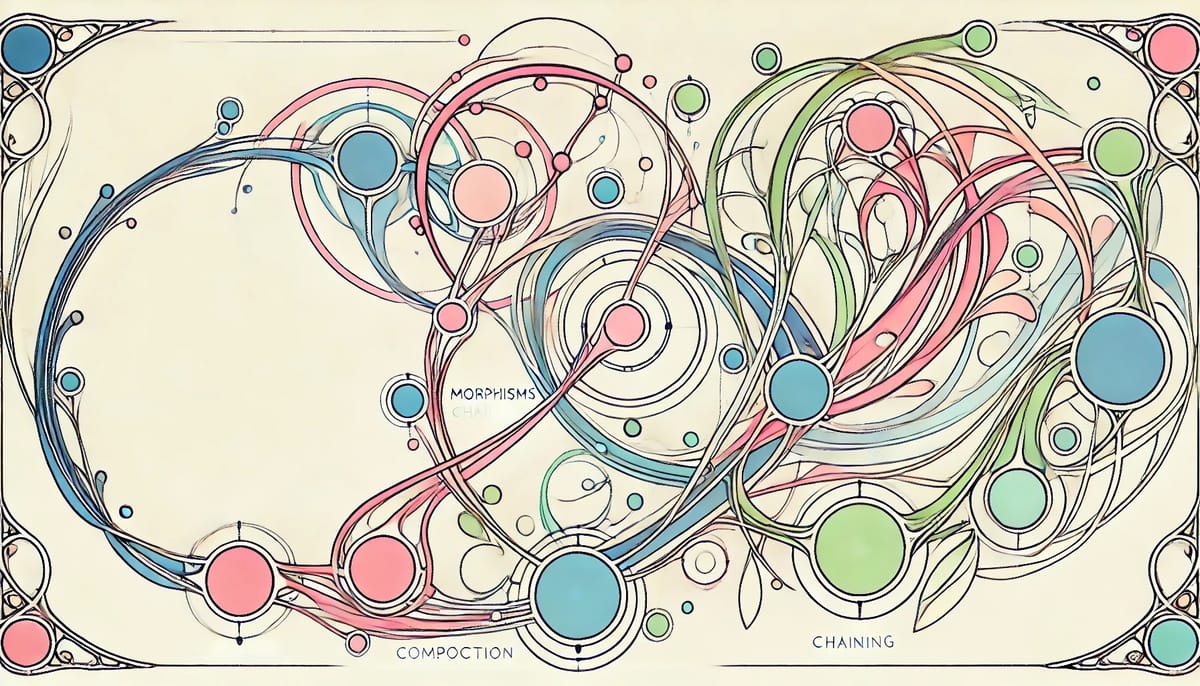
A Kleisli category provides a way to encapsulate and compose effectful computations, which is especially appealing if you're familiar with functional programming and monads.
Core Concept
Given a monad $T$ on a category $\mathcal{C}$, the Kleisli category $\mathcal{C}_T$ is constructed as follows:
- Objects: The objects in $\mathcal{C}_T$ are exactly the objects of $\mathcal{C}$.
- Morphisms: Instead of plain functions $f: A \to B$, a morphism in $\mathcal{C}_T$ from $A$ to $B$ is a function $f: A \to T B$ in $\mathcal{C}$. In other words, arrows represent computations that produce a result in $B$ wrapped in the monadic context $T$.
- Identity: For any object $A$, the identity arrow in the Kleisli category is given by the unit of the monad, $\eta_A: A \to T A$.
- Composition: The composition of two Kleisli arrows
$$
f: A \to T B \quad \text{and} \quad g: B \to T C
$$
is defined using the monadic bind (or the monad multiplication $\mu$):
$$
g \circ f = A \xrightarrow{f} T B \xrightarrow{Tg} T^2 C \xrightarrow{\mu_C} T C.
$$
This composition is often referred to as Kleisli composition.
Why It Matters in Functional Programming
- Encapsulation of Effects: In languages like Haskell, where monads are used to manage side effects (e.g., IO, state, exceptions), the Kleisli category provides the abstract mechanism to chain these effectful computations while keeping the functional purity intact.
- Composition: Kleisli arrows make it possible to compose effectful functions in a manner analogous to composing pure functions. Instead of dealing with the boilerplate of unwrapping and re-wrapping the monadic values, Kleisli composition handles it for you.
- Abstraction: It abstracts away the monadic plumbing. When you think in terms of the Kleisli category, you're working at a level where you only consider the "essence" of the computations — how to chain them together — without being bogged down by the specifics of the monad's mechanics.
Reflective Questions
- Interoperability: How might you leverage the Kleisli category when designing a DSL (domain-specific language) that needs to integrate various kinds of side effects?
- Abstraction vs. Implementation: Can you think of scenarios where the clarity provided by the Kleisli category might be compromised by over-abstraction, and how would you address that in your design?
- Alternative Approaches: In your experience with functional programming, what are the trade-offs between using direct monadic composition (i.e., using bind directly) and abstracting your computations in a Kleisli category framework?
The concept of a Kleisli category elegantly marries the abstract foundations of category theory with the practical needs of functional programming, providing both a theoretical and a practical framework for understanding and managing computational effects.
int -> int
. A Kleisli category is where the objects are the same as the original regular cateogry, but the functions (morphisms) are of the type int -> Wrapper[int]
?Exactly. In a Kleisli category for a monad $T$, while the objects remain the same as in the original category, the arrows (or morphisms) change from regular functions $f: A \to B$ to functions $f: A \to T(B)$. Using your example, if we consider the type int
as an object and Wrapper
as the monad $T$, then a Kleisli arrow would indeed have the type:
$$
\texttt{int -> Wrapper[int]}.
$$
Key Points
- Objects Unchanged: The objects are the same as in your base category (here, types like
int
). - Morphisms as Wrapped Functions: Each morphism is a function that takes an input of type $A$ and returns a value of type $T(B)$ (here,
Wrapper[int]
instead ofint
). - Composition via Bind: Instead of composing plain functions, the Kleisli composition takes advantage of the monadic bind (or join) to chain these wrapped computations seamlessly. For two functions:$$
f: A \to T(B) \quad \text{and} \quad g: B \to T(C),
$$the composition is defined as:$$
g \circ f = A \xrightarrow{f} T(B) \xrightarrow{Tg} T(T(C)) \xrightarrow{\mu_C} T(C),
$$where $\mu$ is the monad's multiplication (or flattening operation).
Reflective Thoughts
- Design Implications: How might this abstraction change the way you approach error handling or asynchronous operations in a functional language?
- DSL Construction: Could framing your computations in terms of Kleisli arrows lead to more modular designs in your domain-specific language, and what trade-offs might arise from such an abstraction?
- Overhead Consideration: While the abstraction is elegant, in what scenarios might the additional wrapping and unwrapping introduce inefficiencies, and how might you mitigate them?
This perspective allows you to manage effects while still reasoning compositionally about your functions, which is a powerful tool in functional programming.
g . f
You have to do something different. and that's a Kleisli arrow? how does it work exactly?Exactly—standard function composition doesn’t directly apply because you’re now working with functions that return wrapped values. In the Kleisli category, you “compose” these functions using the monad’s bind operation (or equivalently, its multiplication/flattening operation).
How Kleisli Composition Works
Suppose you have:
- $f: A \to T(B)$
- $g: B \to T(C)$
Instead of composing them directly as $g \circ f$, you define their Kleisli composition $g \star f$ (often written simply as $g \circ f$ in the context of Kleisli categories) by the following process:
- Apply $f$ to an input $a \in A$:
You get $f(a)$ which is of type $T(B)$. - Bind $f(a)$ to $g$:
The bind operation (often denoted as \texttt{>>=}) takes the wrapped value $f(a)$ and a function $g: B \to T(C)$, and applies $g$ to the unwrapped value $b$ (inside the context of $T$) to yield $T(C)$.
In formula terms:
$$
(g \star f)(a) = f(a) \text{ >>= } g.
$$
Alternatively, if you want to express it using the monad's functorial action and flattening (denoted by $\mu$):
$$
(g \star f)(a) = \mu_C\Big( T(g)\big( f(a) \big) \Big).
$$
Here, $T(g)$ applies the function $g$ inside the context $T$ (so it goes from $T(B)$ to $T(T(C))$), and then $\mu_C$ flattens $T(T(C))$ to $T(C)$.
Exactly. The bind operation—often known as $flatMap$ in many programming languages—is precisely what you described. It takes a wrapped value (say, a value of type $Wrapper[A]$) and a function that operates on the unwrapped value (of type $A \to Wrapper[B]$), then applies the function and “flattens” the resulting nested wrapper (i.e., from $Wrapper[Wrapper[B]]$ back to $Wrapper[B]$).
How It Works
Consider:
- A wrapped value: $m: Wrapper[A]$
- A function: $f: A \to Wrapper[B]$
Using $bind$ (or $flatMap$), you perform:
m >>= f -- or equivalently: m.flatMap(f)
This operation:
- Extracts the value from $m$.
- Applies the function $f$ to that value, resulting in $Wrapper[B]$.
- Flattens the result so that you don't end up with a nested $Wrapper[Wrapper[B]]$.
Summary
- Bind/FlatMap: Both names refer to the same operation.
- Single Layer of Wrapping: It ensures that even after applying functions that return wrapped values, the overall structure remains a single layer (e.g., $Wrapper[B]$).
Reflective Considerations
- Error Handling: How do you think using $bind$ might simplify managing errors in your code, especially compared to manually handling nested structures?
- Readability vs. Abstraction: While $flatMap$ abstracts the unwrapping/flattening, can you recall any instances where this abstraction might make the underlying data flow less obvious? How did you address that?
$bind$ is fundamental to working with monads, allowing you to chain computations without drowning in nested contexts.
(g⋆f)(a)=f(a) >>= g
Can you write that in terms of a flatmap?Yes, exactly. In a language that provides a $flatMap$ method, the Kleisli composition can be written as:
$$
(g \star f)(a) = f(a).\texttt{flatMap}(g)
$$
Here, $f(a)$ produces a wrapped value, and $flatMap$ applies the function $g$ to the unwrapped value, flattening the result so you end up with a single layer of wrapping.
- How might leveraging such compositional techniques help streamline the handling of effects (like error handling or asynchronous computations) in your current projects?
int -> Wrapped[int]
and g is Wrapped[int] -> Wrapped[int]
?Not quite. In the Kleisli framework, both functions operate on the raw values and then wrap them. More precisely:
- $f$ is a function of type:
$int \to Wrapped[int]$
(It takes an $int$ and returns a wrapped $int$.) - $g$ should also be a function of type:
$int \to Wrapped[int]$
(It takes an $int$ and returns a wrapped $int$.)
When you compose them using Kleisli composition, you write:
$$
(g \star f)(a) = f(a).\texttt{flatMap}(g)
$$
Here’s what happens:
- $f$ takes an $int$ $a$ and returns a value of type $Wrapped[int]$.
- $\texttt{flatMap}$ then extracts the inner $int$ from this wrapped value and applies $g$ to it.
- $g$ produces another $Wrapped[int]$, and $\texttt{flatMap}$ ensures that the final result remains a single layer of wrapping.
If $g$ were instead of type $Wrapped[int] \to Wrapped[int]$, you would be applying it directly to a wrapped value, which is not how Kleisli arrows work. The key is that $g$ expects a raw $int$ (the unwrapped value), not a wrapped one.
Reflective Consideration
- How might ensuring that $g$ has the correct type (i.e., unwrapped input) influence the way you design your function compositions when dealing with asynchronous or error-handling computations?
Exactly. In the Kleisli category, composing two arrows is performed using the $flatMap$ (or $bind$) operator. When you have:
- $f: \texttt{int -> Wrapped[int]}$
- $g: \texttt{int -> Wrapped[int]}$
Their composition is defined by:
$$
(g \star f)(a) = f(a).\texttt{flatMap}(g)
$$
In this sense, $flatMap$ acts as the composition operator, allowing you to chain these functions while handling the wrapping and unwrapping automatically.
Yes, they're essentially the same operation in this context. In many functional programming libraries and languages, $flatMap$ and Elm's $andThen$ serve the same purpose: they allow you to chain computations that return a wrapped value (like $Maybe$ or $Result$) without nesting.
- Type Signature:
Both $flatMap$ and $andThen$ typically have a similar type signature. For a monad $M$, it often looks like:
$$
(a \to M, b) \to M, a \to M, b
$$
In Elm, for example, $andThen$ for $Maybe$ or $Result$ follows this pattern—taking a function that maps an unwrapped value to a wrapped one, and then chaining it onto an existing wrapped value. - Conceptual Role:
They both abstract the pattern of taking a wrapped value, applying a function to the unwrapped content, and flattening the result to maintain a single layer of wrapping. - Reflective Consideration:
When transitioning between languages or libraries, do you think the naming differences (e.g., $flatMap$ vs. $andThen$) impact your mental model of chaining computations, or do they merely reflect different community conventions?
In summary, in this context, $flatMap$ and $andThen$ are functionally equivalent and serve as the composition operator in their respective settings.
Exactly. The Writer monad is a classic example where, instead of simply returning a value, you return a tuple—typically something like $(\text{value}, \text{log})$. Here’s how it aligns with the Kleisli category concept:
- Wrapped Value: With the Writer monad, the wrapped type is something like $ \texttt{Writer a} \approx (a, \text{log}) $. This “wraps” the usual value with additional information (e.g., logging, state, etc.).
- Kleisli Arrows: When you work with the Writer monad, your functions become of the type $ f: A \to \texttt{Writer B} $. They take a plain value and return a pair containing the result along with the accumulated log. This is analogous to having functions $ A \to T(B) $ in a Kleisli category, where $ T $ is your Writer monad.
- Composition via flatMap/andThen: By using the $ \texttt{flatMap} $ (or $ \texttt{andThen} $ in Elm) operation, you can compose these functions without having to manually manage the additional log information. The monad’s bind operation ensures that the logs from multiple computations are combined appropriately while preserving the single-layer structure of the Writer monad.
Reflective Considerations
- Design: How might using a Writer monad simplify debugging or auditing computations in your projects by automatically accumulating logs as you chain functions together?
- Abstraction: In scenarios where additional context or side effects need to be tracked alongside pure computations, what are some trade-offs between using a Writer monad and other approaches (such as explicit logging)?
This approach—lifting your computations into a context where they return wrapped values—is central to many functional programming patterns and is one of the key insights behind using Kleisli categories to manage effects elegantly.
Exactly. A Reader monad (sometimes called the Environment monad) is designed to thread a shared environment or context through a series of computations, without you needing to pass it explicitly every time. Let's break it down in the context of Kleisli categories and the concepts we've discussed:
The Reader Monad in a Nutshell
- Basic Idea:
A Reader monad encapsulates functions that depend on some environment $r$. Instead of writing functions with an extra parameter for the environment (e.g., $f: A \times r \to B$), you define functions that take an $A$ and return a function $r \to B$. That is, the type becomes:
$$ f: A \to (r \to B) $$
This is equivalent to writing $f: A \to \text{Reader } r,B$ where $\text{Reader } r,B$ is the wrapped type. - Kleisli Category for the Reader Monad:
In the Kleisli category for the Reader monad, the objects remain the same (types like $A, B$, etc.), but the morphisms are functions that return a value in the Reader monad, i.e., $A \to (r \to B)$.
How Composition Works in the Reader Monad
Suppose you have two functions:
- $$ f: A \to (r \to B) $$
- $$ g: B \to (r \to C) $$
Their Kleisli composition (using the bind operation, which for the Reader monad acts as \texttt{flatMap}) is defined as:
$$ (g \star f)(a) = f(a) ;\texttt{flatMap}; g $$
For the Reader monad, the bind operation works like this:
- Apply $f$:
Given an input $a \in A$, $f(a)$ is a function $r \to B$. - Thread the Environment:
For a given environment $r$, you compute $b = f(a)(r)$. - Apply $g$:
Then you compute $g(b)$, which is another function $r \to C$. - Final Composition:
The overall composition yields a function $r \to C$ that is defined as:
$$ (g \star f)(a)(r) = g\bigl(f(a)(r)\bigr)(r). $$
Here, the environment $r$ is implicitly passed along through both computations.
Reflective Considerations
- Implicit Context:
How do you feel about the trade-off of having an implicit environment threaded through computations versus passing it explicitly? Does this abstraction help clarify your code's intent or hide too much detail? - Error Handling and Testing:
Since the environment is handled implicitly, what strategies might you employ to ensure that changes in the environment don't lead to subtle bugs or unintended side effects? - Interoperability:
When integrating with functions that require an explicit environment, how might you leverage the Reader monad to encapsulate that dependency cleanly?
Summary
The Reader monad lifts your ordinary functions into a context where they automatically receive an environment, which is precisely the idea behind lifting functions into a Kleisli category. Here, the arrows are not simply $A \to B$ but $A \to (r \to B)$, and composition via the bind (or \texttt{flatMap}) operation ensures that the shared environment is consistently threaded through your computations.
This abstraction not only simplifies the function signatures by eliminating repetitive environment parameters but also cleanly separates the concerns of environment management from the core business logic.
Exactly. In the Reader monad, the wrapped value is essentially a computation that, when provided an environment, returns a result. The "wrapper" is that function $r \to a$, which implicitly carries along the environment $r$.
When you have functions that operate on these results within the Reader context — say, functions of type $A \to (r \to B)$ — you don't want to manually extract the environment and then reapply it. Instead, you use Kleisli arrows (with bind/flatMap) to chain these computations. This composition ensures that the environment is threaded through automatically, so you can manipulate the result while keeping the context intact.
For example, if you have:
- $f: A \to (r \to B)$
- $g: B \to (r \to C)$
Their Kleisli composition is:
$$
(g \star f)(a)(r) = g\big(f(a)(r)\big)(r)
$$
This way, you can chain the functions without ever needing to "unwrap" the environment manually. It all happens seamlessly under the hood via the bind operation.
Reflective Considerations
- How might this implicit threading of the environment simplify your code compared to explicitly passing the environment to every function?
- In what situations could this abstraction hide complexities, and how would you design tests to ensure that the environment is being threaded correctly?
- Are there cases where you might prefer an explicit approach over using the Reader monad, especially when debugging complex environment-dependent logic?
This approach highlights the power of Kleisli categories in managing context or side effects — in this case, the shared environment — without sacrificing the clarity of your code.
Kleisli categories provide a structured way to model effectful computations by reinterpreting functions so that they return values in a computational context (i.e., wrapped in a monad). In a Kleisli category, instead of having a plain function $f: A \to B$, you work with arrows of the form $f: A \to T(B)$, where $T$ is a monad that encapsulates some effect (like state, logging, IO, etc.). This means you can chain effectful computations using the monad’s bind (or flatMap) operation without manually handling the effectful wrapping and unwrapping.
$$
\textbf{Encapsulation of Effects:}
$$
Kleisli categories abstract away the explicit handling of effects. By lifting computations into the monad $T$, you can compose them seamlessly while preserving the effectful context. This abstraction allows you to reason about effectful functions as if they were pure, while the monad takes care of the side effects.
$$
\textbf{Composition of Effectful Functions:}
$$
In the Kleisli framework, composition is redefined so that given $f: A \to T(B)$ and $g: B \to T(C)$, their composition is:
$$
(g \star f)(a) = f(a) \text{ flatMap } g
$$
This operation ensures that the effects encapsulated by $T$ are properly combined (e.g., logs are accumulated, state is threaded, etc.).
$$
\textbf{Relationship to Algebraic Effects}
$$
Algebraic effects are an alternative (and in some ways, more flexible) approach to modeling effects. Here’s how they relate to the Kleisli (monadic) approach:
- Declarative Specification of Effects:
Algebraic effects allow you to declare effects via operations and then later interpret or "handle" these effects using effect handlers. This separates the description of the effectful operations from their implementation, leading to a highly modular design. - Free Monads and Kleisli Categories:
Many algebraic effect systems can be modeled using free monads. The free monad construction gives you a way to represent effectful computations as data structures. Since free monads are monads, they inherently have an associated Kleisli category. In this sense, Kleisli categories are indirectly related to algebraic effects through the representation of algebraic effect operations as free monads. - Flexibility in Handling Effects:
While Kleisli categories give you a framework for composing effectful functions under a specific monad, algebraic effects often provide more fine-grained control by letting you define multiple handlers for the same effect or compose effects in different ways. This can be seen as a more general or flexible approach compared to committing to a particular monadic structure.
$$
\textbf{Reflective Considerations:}
$$
- Abstraction vs. Control:
How do you balance the abstraction provided by Kleisli composition with the need for explicit control over effect handling in your projects? - Interchangeability and Modularity:
Can you envision a scenario where you start with a monadic (Kleisli) design and then migrate to an algebraic effect system to achieve more modular handling of effects? What would be the trade-offs? - Error and Effect Handling:
Given that algebraic effects allow you to separate effect declaration from interpretation, in what cases might this provide an advantage over traditional monadic approaches (and vice versa)?
In summary, Kleisli categories offer a categorical framework for modeling and composing effectful computations via monads, while algebraic effects provide a more modular approach by separating effect specification from interpretation. Both frameworks aim to manage side effects cleanly, but they differ in their abstraction and control over effect handling.