Effects as Protocols and Context as Agents
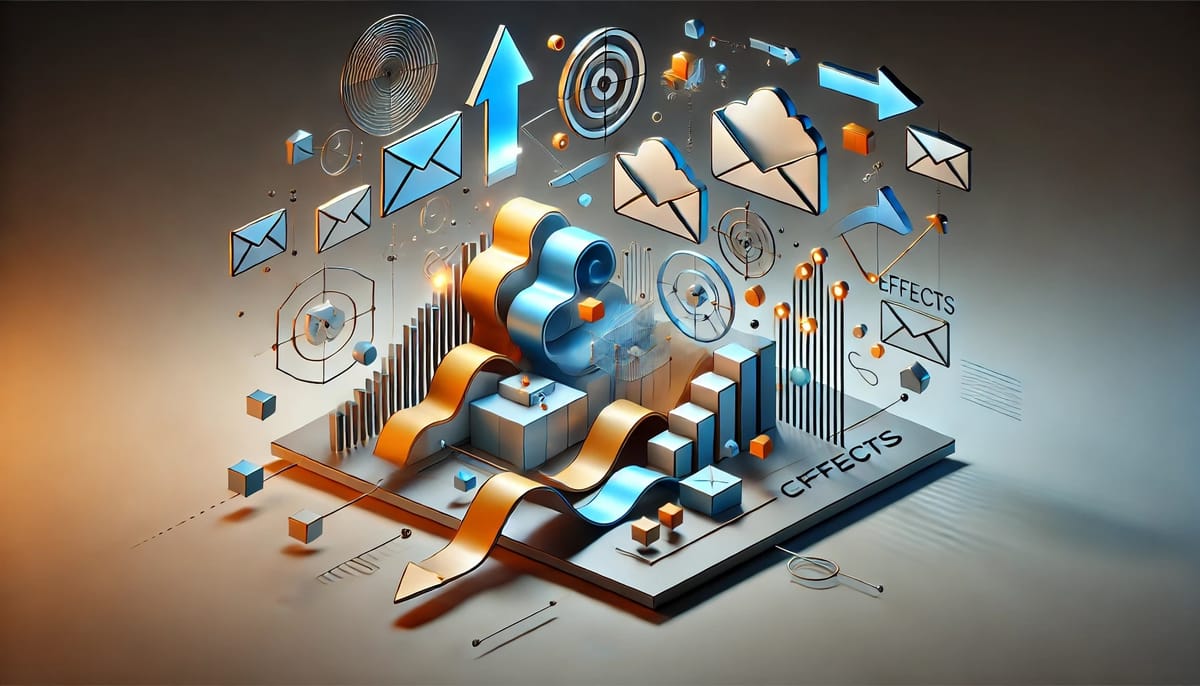
Target audience: mainstream developers that have done a little bit of functional programming.
Introduction
What if we thought of effects not as mysterious side operations but as conversations with an agent managing our program's context? This reframing offers a powerful perspective on how effects unify various programming paradigms, from state and exceptions to closures and continuations. By viewing effects as communication protocols with the program's context, we gain not only a more structured mental model but also the tools for building modular, extensible interpreters.
Effects—often thought of in terms of state changes or I/O—are at the core of how programs interact with their environment. But effects are more than just side operations; they are the mechanisms by which a program and its context communicate. This post dives into the concept of effects as protocols and explores why this perspective is foundational for extensible interpreters and modular programming. Whether you're designing compilers, writing functional code, or architecting distributed systems, understanding effects through this lens will elevate your approach to program design.
What Are Effects, Really?
At its core, an effect is an interaction with the context. Context here means anything external to a specific computation: it could be state, the file system, a database, or even the set of variables in scope. When a program performs an effect, it sends a request to this context and receives a response. This interaction is bidirectional, forming a protocol between the program and the context.
Let’s clarify this with an example. Consider a program that manages state:
get :: State Int Int
put :: Int -> State Int ()
The get
operation requests the current state, and put
updates it. These operations don’t directly manipulate state; instead, they describe what the program wants to do. The actual state management happens in a handler that processes these requests and replies with results. This separation between effect description and effect handling is what makes the protocol explicit.
Effects, therefore, are not limited to observable changes like I/O. They encompass any interaction with the program's context, including resolving variable bindings or managing closures. Whether we treat something as an effect often depends on what level of abstraction we’re working at. For example, accessing a variable might not feel like an effect when evaluating a function, but it’s undeniably a contextual interaction when designing an interpreter.
Effects as Protocols: The Communication Model
The most useful way to understand effects is as protocols. The program sends requests to the context, and the context responds. This interaction formalizes what the program needs to proceed, and how the context provides that information. By treating effects as protocols, we gain a clear structure for reasoning about complex computations.
Let’s break this down using Oleg Kiselyov’s extensible interpreter as an example. In this model, effects are represented as requests. For instance, resolving a variable might be represented by:
Req (ReqVar v) k
Here:
ReqVar v
is the request to resolve the variablev
.k
is the continuation, or callback, that specifies what to do once the variable is resolved.
Similarly, creating a closure (a function with its environment) is modeled as:
Req (ReqClosure v body) k
This protocol treats the context as an agent responsible for handling these requests. For example:
- If a variable
v
exists in the current environment, the context retrieves its value and passes it to the continuationk
. - If the request is for a closure, the context captures the current environment and returns a function bundled with this environment.
Why Protocols Matter:
- Simplified Reasoning: By abstracting the mechanics of effects into a protocol, the program’s logic becomes more explicit and easier to follow.
- Modularity: Effects can be implemented or interpreted differently without changing the program’s logic. For example, state effects could be handled in memory or by a database—the protocol remains the same.
- Recursiveness: Protocols naturally support recursive computations where subexpressions may themselves involve effects.
Why Formulate Effects as Protocols?
One of the key motivations for formulating effects as protocols is the need for extensible interpreters. In traditional interpreters, adding new features like state or exceptions often requires invasive changes throughout the evaluation engine. Every piece of the interpreter must be updated to support the new feature. This tightly couples the interpreter’s logic to its features, making it harder to maintain and extend.
Treating effects as protocols solves this problem by isolating the description of an effect from its implementation. Each effect is a request that can be handled independently by a handler. For example, to add state to an interpreter, you might define:
get :: StateReq
put :: Int -> StateReq
And implement a handler like this:
handleState :: State -> Comp -> Comp
handleState s (Done x) = Done x
handleState s (Req (ReqState Get) k) = handleState s (k s)
handleState s (Req (ReqState (Put newState)) k) = handleState newState (k ())
This handler processes state requests (Get
and Put
) without touching the rest of the interpreter. By defining effects as protocols, we decouple their handling from the core evaluation logic, enabling modular and extensible designs.
Other advantages of this approach include:
- Composability: Handlers for different effects can be combined easily. For example, you might handle state and logging together.
- Reusability: Handlers can be reused across different interpreters or systems. For example, a state handler could back state with a file system, in-memory variables, or a database.
Protocols also allow for dynamic extension, where new effects can be added without modifying existing code. This flexibility is particularly valuable for building domain-specific languages or experimental runtime systems.
Effects and Closures: A Deep Dive
Closures are one of the most elegant features of modern programming languages, enabling functions to carry their surrounding environment wherever they are invoked. However, from the perspective of effects as protocols, closures reveal a fascinating interaction between program and context. Closures inherently involve capturing context, which makes them a prime example of contextual interactions treated as effects.
When a closure is created, it captures the current environment, which maps variable names to their corresponding values. This environment is then bundled with the closure and carried along wherever the closure goes. In terms of effects, closure creation can be modeled as a request:
Req (ReqClosure v body) k
Here:
ReqClosure v body
represents the operation of creating a closure for the parameterv
with the function bodybody
.k
is the continuation that specifies what to do after the closure is created.
The handler for this request performs two critical tasks:
- Captures the current environment (a context interaction).
- Constructs a new function (
DCFun
) that extends the environment with a new binding when the closure is invoked.
Consider the following code:
let f = \x -> \y -> x + y in
let g = f 5 in
g 3
When f
is evaluated, the interpreter generates a closure that captures the current environment, binding x
. When f
is applied to 5
, the resulting closure for g
further captures x = 5
. Finally, invoking g
with 3
resolves both x
and y
in the extended environment, computing 5 + 3 = 8
.
Managing the Environment
The environment—a mapping of variable names to values or closures—is central to how closures interact with their context. The handler maintains the environment and updates it dynamically:
- Capturing Context: When creating a closure, the current environment is saved and frozen.
- Extending Context: When a closure is applied, the environment is extended with new variable bindings for the closure’s parameters.
- Resolving Variables: When a variable is referenced, the handler looks it up in the current environment.
This recursive interaction ensures that closures remain self-contained and predictable, with no unintended interference between different parts of the program. The environment’s immutability—each extension creates a new environment rather than modifying the old one—further ensures that closures behave consistently.
Code Walkthrough: Nested Closures
Let’s walk through a detailed example of nested closures:
let f = \x -> \y -> x + y in
let g = f 2 in
g 3
Create f
:
Req (ReqClosure "x" (\y -> x + y)) Done
- The handler creates a closure:
DCFun $ \x -> handleVar ("x", x : env) (\y -> x + y)
Assign g = f 2
:
- Apply the closure
f
to2
:
app (DCFun $ \x -> ...) 2
- The environment is extended:
("x", 2)
. - A new closure is returned:
DCFun $ \y -> handleVar ("y", y : "x", 2 : env) (x + y)
Invoke g 3
:
- Apply the closure
g
to3
:
app (DCFun $ \y -> ...) 3
- The environment is extended:
("y", 3), ("x", 2)
. - Resolves
x + y
as2 + 3 = 5
.
This example demonstrates how closures, environments, and effects intertwine to create a modular and extensible mechanism for managing contextual interactions.
Why Effects Are Perspective-Dependent
One of the fascinating aspects of effects is how their interpretation shifts based on the lens through which we view computation. Effects can be considered context interactions, but what we choose to define as an effect depends heavily on our goals and focus. This perspective shapes how we design, implement, and reason about our systems.
Viewing Effects in Context
At the lowest level, every interaction with the context can be treated as an effect. For example:
- Accessing a variable involves querying the current environment.
- Creating a closure involves capturing a surrounding context of variable bindings.
From this viewpoint, even basic operations like variable lookups are contextual interactions, and thus, effects.
However, at a higher abstraction level, these interactions may not be regarded as effects. For instance, in functional programming, accessing a variable is often treated as a pure operation because it is deterministic and fully encapsulated within the program logic. The same operation could be seen as an effect in a compiler where performance optimizations require considering the cost of variable access.
Practical Examples of Perspective
Expression Evaluation:
- When focusing on the result of a computation, the emphasis is on observable side effects, like state changes, I/O operations, or exceptions. Variable lookups and function calls are often abstracted away as pure operations.
Performance Optimization:
- Compilers, on the other hand, must treat even basic operations like variable lookups as effects. For example, accessing a local variable versus a global variable might differ in cost, making such distinctions crucial during optimization.
System Design:
- When building extensible interpreters or domain-specific languages, effects are explicitly modeled to simplify reasoning and modularity. Here, even the act of resolving a variable or managing a closure may be treated as an effectful operation.
Effects as Abstraction Boundaries
The key insight is that effects serve as abstraction boundaries. They let us compartmentalize the parts of a system we want to reason about explicitly while abstracting away others. By treating context interactions as protocols, we focus on how different components communicate, leaving implementation details to handlers or the runtime.
This abstraction also enables different degrees of modularity. For instance:
- Algebraic effects allow the separation of effect declarations from their implementations.
- Protocol-based systems like event-driven architectures encapsulate interactions between components.
Whether something is an effect or a pure operation depends on where we draw the boundary—and this boundary is determined by the system’s goals.
Why Monads Naturally Arise
Monads have long been the cornerstone for managing effects in functional programming. They provide a structured way to encapsulate context interactions while preserving equational reasoning and modularity. What makes monads particularly elegant is how naturally they emerge from the need to manage sequential context interactions.
Sequencing Interactions
At their core, effects involve sequencing: one interaction with the context depends on the result of a previous interaction. Monads formalize this sequencing by encapsulating both the context and the continuation—what happens next—into a single abstraction.
Take the state monad as an example:
newtype State s a = State { runState :: s -> (a, s) }
instance Monad (State s) where
return x = State $ \s -> (x, s)
(State f) >>= g = State $ \s -> let (a, s') = f s
in runState (g a) s'
This structure accomplishes two key things:
- Context Management: The state (
s
) is threaded through each computation. - Continuation Chaining: Each computation produces a result (
a
) and an updated context (s'
), which are passed to the next computation (g
).
Monads as Protocols
Monads encapsulate context interactions by defining two operations:
return
: Embeds a value into the monadic context.>>=
(bind): Sequences computations by chaining their interactions with the context.
This mirrors the protocol-like structure of effects:
- A computation describes what it needs from the context (e.g.,
get
,put
,throw
). - The monadic structure determines how these requests are sequenced and how their results flow through subsequent computations.
Algebraic Effects vs. Monads
While monads are powerful, they intertwine the description of effects with their sequencing. Algebraic effects take this one step further by decoupling these concerns:
- Monads: Encapsulate both the description and the sequencing of effects.
- Algebraic Effects: Separate the declaration of effects (e.g.,
get
,put
) from their interpretation (handlers).
This separation allows for greater composability and modularity. For example, an algebraic effect system can handle state, exceptions, and I/O independently, combining them only where necessary.
Monads in Practice
Monads are widely used in functional programming languages like Haskell because they:
- Preserve Equational Reasoning: Monads enforce explicit sequencing, making it easier to reason about dependencies between computations.
- Encourage Modularity: Different monads (e.g.,
State
,Reader
,IO
) can encapsulate distinct types of effects, enabling reusable and composable abstractions. - Unify Context Interactions: Monads provide a consistent interface for describing and managing effects, regardless of their nature.
By managing effects through monads, programmers gain control over the flow of context interactions, ensuring that programs remain predictable and maintainable.
Challenges and Counterarguments
While the perspective of effects as context interactions is powerful, it is not without challenges. There are practical limitations and critiques, especially when it comes to composability, reasoning, and implementation.
Composability Issues
One of the most significant challenges with effects is composability. While algebraic effects and monads provide frameworks for managing effects, some effects do not compose cleanly:
- Non-Commutative Effects: Certain effects, like state and continuations, interact in non-intuitive ways. For example, combining
State
andCont
(continuations) can lead to subtle bugs because their interactions depend on the order of operations. - Nested Effects: Handling multiple layers of effects (e.g., state within I/O within exceptions) can introduce significant complexity, especially when effects need to interleave.
Observable Effects
Observable side effects, like I/O, present unique challenges for reasoning and modularity:
- Equational Reasoning: Observable effects break the ability to substitute expressions freely because the order of operations matters.
- Encapsulation: Unlike algebraic effects, observable effects often require tight coupling with the runtime, making them harder to abstract and test.
Broader Critiques
Some critics argue that treating all context interactions as effects can dilute the concept of effects. For example, resolving a variable in a closure might be seen as "too low-level" to warrant modeling as an effect in most practical systems. The balance between theoretical purity and practical applicability remains an ongoing discussion.
Practical Implications for Programmers
Understanding effects as protocols has profound implications for how we design, implement, and reason about programs. By explicitly modeling effects, we gain modularity, clarity, and control over program behavior.
How to Think About Effects
Explicit Boundaries:
- Treat effects as abstraction boundaries. Anything crossing a boundary—whether state, I/O, or variable lookup—should be modeled explicitly to ensure clarity and modularity.
Protocol Design:
- Design effects as protocols between your program and its context. For example, state effects might describe "get" and "put" operations, while exceptions might define "throw" and "catch" operations.
Use Handlers:
- Handlers centralize the implementation of effects, making it easier to modify or extend their behavior without disrupting the program’s logic.
Language Design Lessons
Many modern programming languages incorporate ideas from this perspective:
- Haskell: Uses monads to manage effects, providing explicit sequencing and control.
- Koka: Emphasizes algebraic effects, decoupling effect declaration from interpretation.
- Elm: Treats effects as explicit commands (
Cmd Msg
), which are passed to the runtime for execution.
Everyday Programming
For everyday programmers, this perspective encourages a disciplined approach to managing complexity:
- Use monads or algebraic effects to encapsulate and isolate context interactions.
- Model effects explicitly in interfaces to make dependencies clear.
- Leverage handlers to modularize and extend behavior as your system grows.
Conclusion
Effects are best understood as protocols for interacting with context. This perspective provides a unifying lens through which we can manage state, I/O, exceptions, and even closures. By treating effects explicitly, we enable modularity, extensibility, and clearer reasoning about program behavior.
From designing compilers to writing functional programs to building scalable systems, the key insight is that effects are tools for abstraction. Whether implemented as monads, algebraic effects, or something else entirely, they provide a structured way to manage the interplay between a program and its context.
Understanding effects through this lens doesn’t just make you a better programmer—it fundamentally changes how you think about computation.